Git Tagging Strategies: Versioning Releases Effectively
Prerequisites:
- Git Config Deep Dive: Managing SSH Keys and Multiple SSH Keys with ssh_config
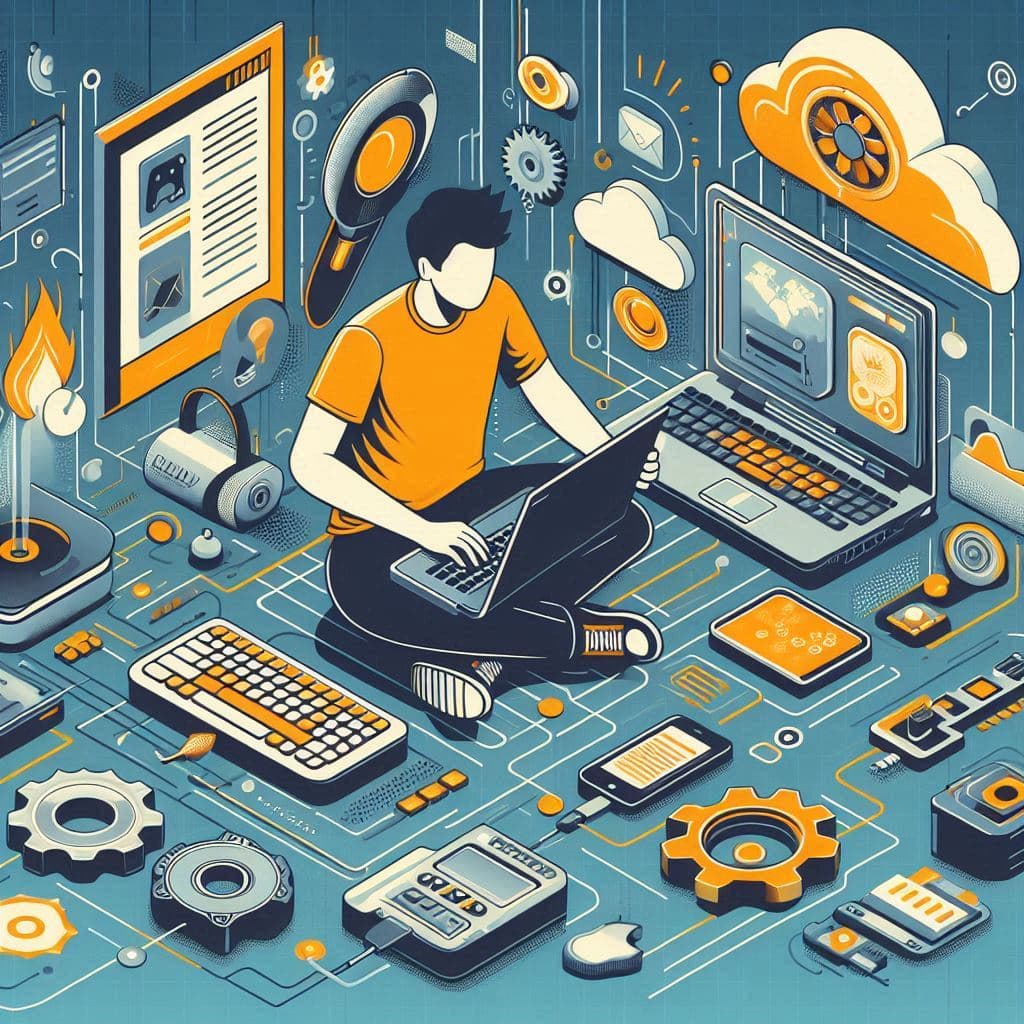
Git Tagging Strategies: Versioning Releases Effectively
Tags in Git are used to mark specific points in history, typically for releases. Effective tagging strategies ensure consistency and clarity in versioning. In this blog, we’ll explore lightweight vs annotated tags, semantic versioning, and automating tagging workflows.
Table of Contents
- Types of Tags
- Semantic Versioning
- Creating and Managing Tags
- Automating Tagging with Scripts
- Exercise: Implementing a Tagging Strategy
Types of Tags
Lightweight tags: Simple pointers to commits. Annotated tags: Full objects with metadata (author, message, date).
Semantic Versioning
Follow the MAJOR.MINOR.PATCH
convention:
- MAJOR: Breaking changes.
- MINOR: Backward-compatible features.
- PATCH: Backward-compatible bug fixes.
Creating and Managing Tags
Create a lightweight tag:
git tag v1.0.0
Create an annotated tag:
git tag -a v1.0.0 -m "Release version 1.0.0"
Push tags to the remote repository:
git push origin --tags
Automating Tagging with Scripts
Write a script to increment version numbers:
#!/bin/bash
version=$(git describe --tags --abbrev=0)
major=$(echo $version | cut -d. -f1)
minor=$(echo $version | cut -d. -f2)
patch=$(echo $version | cut -d. -f3)
new_version="$major.$minor.$((patch+1))"
git tag -a $new_version -m "Release version $new_version"
git push origin --tags
Exercise: Implementing a Tagging Strategy
Practice tagging:
- Create lightweight and annotated tags for a repository.
- Push tags to the remote repository.
- Write a script to automate semantic versioning.
Coming Up Next
In the next part of this series, we’ll explore signing commits and tags with GPG keys to ensure trust and integrity in your code.
Part 20 of 24 in Git Mastery Series: From Beginner to Expert
All Posts in This Series
1. Introduction to Git: What is Version Control?
2. Initializing a Repository and Making Your First Commit
3. Branching and Merging in Git
4. Resolving Merge Conflicts in Git
5. Advanced Git Commands: Cherry-Picking and Interactive Rebase
6. Git Hooks and Automation: Streamlining Workflows
7. Git Workflows and Best Practices: Streamlining Collaboration
8. Debugging with Git: Bisect and Blame
9. Customizing Git: Aliases and Configuration
10. Mastering Git Diff: Analyzing Changes and Advanced Use Cases
11. Common Git Issues and Solutions: Troubleshooting Like a Pro
12. Understanding Git Internals: How Git Works Under the Hood
13. Mastering Git Submodules: Managing Dependencies and Modular Projects
14. Advanced Git Branch Management: Sorting, Pruning, and Deleting Branches
15. Git Reflog Deep Dive: Recovering Lost Commits and Understanding Git’s Safety Net
16. Disaster Recovery with Git: Restoring Corrupted Repositories and Lost Objects
17. Git and Open Source Contributions: Best Practices for Collaborative Development
18. Git Behind Firewalls and Proxies: Overcoming Connectivity Challenges
19. Git Config Deep Dive: Managing SSH Keys and Multiple SSH Keys with ssh_config
20. Git Tagging Strategies: Versioning Releases Effectively
21. Git Security and Signing Commits: Ensuring Trust and Integrity
22. Git and CI/CD Integration: Automating Workflows for Continuous Delivery
23. Git Patch Management: Sharing Changes Without Pushing
24. Partial Clones and Sparse Checkouts: Optimizing Large Repositories